My name is Michael Fritzius, and I'm a computer programmer. Currently I work at Exegy, which is a company specializing in development and support of electronic ticker plants for the use of high frequency trading in the stock market. As a Quality and Performance Engineer, my job is to troubleshoot the ticker at many levels, both at the hardware and software side. The job also includes creating new tests and modifying existing tools as needed.
Previously I worked at Control Microsystems as a lead developer, and focused mainly on embedded code for different projects. I programmed microchips that interface with hardware. Occasionally, I would create a graphic user interface (GUI) that communicated with hardware in order to do data analysis. At the time, there were only two of us comprising the company--myself and the boss, whose specialty was Electrical Engineering and Design. Between the both of us there wasn't a project undertaken that we couldn't handle. I worked there from October 2006 to May 2009, and helped develop a wide variety of industrial controls.
This site was created to serve as a hub for various projects that I'm working on. It seems like it'd be better to have them all listed in one place instead of having them strewn all over the internet.
To the right, you'll find the section showing which projects are currently being worked on. Clicking on whatever is in the box will take you immediately to that project listing so you can stay up-to-date on what progress has been made.
Also, this page will serve as a place for any potential employers to find out about my skillset--so if you're looking for a developer with a strong desire to learn and a creative streak, then look no further.
That sounded really corny. Sorry.
Anyway, if you would like to take a look at my resume, it will be found below the projects section.
The current page is going to be the only page for this blog, since all that's needed is to update this page when projects change status, or new ones are added. Click on any of the sub-blog titles to view a project.
Power Grid Game
See Latest Updates
I'm not exactly sure what else to call this, seeing as I just started slamming out code before coming up with a catchy name. I wanted to work on something a little fun in the interim period before things get busy again, plus it sharpens my skills with graphics programming.
The graphics language used is OpenGL, and I'm using 3D perspectives plus masking to generate the game board. It could be done in 2D, but I'm not as familiar with 2D as I'd like--plus I believe it's possible to optimize code enough that the difference between 2D and 3D will be minimal.
Basically, the goal of the game is to rotate game pieces to create a contiguous path from one side of the game board to the other. The game board is populated with different colored pieces, staggered diagonally. Left clicking a piece will rotate the surrounding six pieces counterclockwise, and right clicking will rotate them clockwise. Each piece color will have a unique path on it, and it's the player's job to get the proper pieces in line to complete the path. There is a time limit, and if you can't make a path in time, the game's over.
Some ideas for the different piece types:
Occasionally, "wild" pieces will show up with a universal path that will connect in any direction.
"Void" pieces will just get in the way, not allowing the player to use it to finish a path.
"Bomb" pieces will explode surrounding pieces, clearing out any pieces that get caught up in the blast
"Lock" pieces will prevent any rotation if this piece is one of the six that would have been rotated. These can also be destroyed if in range of a bomb piece.
The screenshot of the game board is pretty rough, so I want to get something a little nicer looking to post here. This project was started around the end of 2008, and seems like it's pretty simple. Hopefully this doesn't take too long. I imagine the bulk of the work will be with the cosmetic stuff rather than the main game engine.
Updates
1-18-09
The picking algorithm is finally settled. The problem was the difference between where OpenGL says a power cell is located and where the mouse says a power cell is located.
OpenGL takes into consideration where an object's physical 3D location is, while a mouse click is just an x-y coordinate on the screen.
Since the perspective of the game board doesn't change, I figured, why not just use the coordinates the mouse provides?
Every cell is of a certain radius. So if I know where all the center points of every cell are located, I can just brute force all 200 cells and figure out which one has the center closest to where I clicked.
So, all the center points are stored along with the color data for each piece. When a click is made, the program runs through all 200 cells and figures out which cell was closest, and picks that cell.
Finally, I can move onto other stuff. Enjoy the screenshot.
1-14-09
This update concerns the particle engine and decision algorithm for creating a path.
The picture to the left shows what I've got for the particles. Each one rotates independently--kind of a minor thing but looks cool, so I'll keep it.
But I can't get sidetracked much on final stage stuff. The way to figure out if a path has been completed is a ton more important.
What I plan on doing is a recursive function that begins with the left side of the board, and checks each of the surrounding 6 pieces to see if they connect. If they do, the search is called again with the focus on the adjacent piece. Whenever a piece is connected that is the 20th piece in that row (the furthest right value for a piece), then a path has been completed.
1-12-09
The latest update involves the picking and rotation algorithm. Picking still needs a little bit of work, because selecting pieces further to the right of the screen makes the algorithm guess wrong most of the time--you have to click on the rightmost edge of the piece for it to pick correctly. Probably there will need to be some floating point calculation done, rather than integer math, to get it perfect.
The rotation algorithm uses whichever piece was selected, and operates on the six surrounding pieces. Looks pretty cool when madly clicking around.
The next step is to fix up the picking algorithm, put in place some error checking (such as not allowing rotation along the edges of the game board), and implementing the particle engine.
I'm amazed how much functionality is already enabled in the particle engine code, and if you're interested, it can be found at NeHe's site here. If you have the ability to download and run the code, you'll notice that all the particles kind of congregate in a little square until they explode outward. This is ideal, since a square that size will conceal the piece, so that it can be "destroyed" and replaced with pieces above. Again, I'd like to make the particles be the color of the piece so that it looks more like fragments of the piece are shooting all over the place. Plus, it just looks flippin cool. This code doesn't use any kind of timer to tell when to draw each frame, which means it's drawn as fast as the computer can handle. That's not so desirable to me because I want to cater to whatever speed machine is running. So in order to optimize this engine, I'll need to incorporate a timer to govern when individual frames can be drawn.
The goal for the particle engine is to create the particles and activate the explosion just when pieces are clicked--not destroy the piece, but showing the particles only.
Also, gearing more toward the game engine itself, I'll have to draw a path on every kind of piece and code how to decide if a path has been completed. I would like to have the path be a different color, like bright blue, whenever it's connected to the left hand side of the game board. This way the player can see where to link up easier. I'll probably have to figure out how to make a tree based on what pieces are connected, and if any piece is on the right hand edge, a path has been completed.
1-6-09
Most OpenGL programs will have an infinite while( ) loop that redraws the screen regardless of what has happened. That's fine for games that usually have something different from the last time the screen was drawn, but for the most part, the screen will look the same unless the user DOES something with the mouse. So, optimizing this program will be a matter of only redrawing the screen whenever the user enacts a change in the game board.
The status right now is the game needs to know how to find out which piece was clicked. Left or right clicking is pretty easy to code in, and rotating the pieces accordingly is a little harder. But, pieces are drawn in every drawing loop, so that's taken care of. Currently, I'm using simple circles as placeholders rather than spending too much time making neat looking pieces.
The whole game needs to have a running timer. I have a piece of code that uses such a timer, and it will be used to time different frames of animation, figuring out how long a player has to create a path, and whatever else is required.
Extras: I would like to incorporate a "path lightning" algorithm and a particle engine to make things look a little snazzier. Of course, I'm not going to start working on these aspects while the main engine is still unfinished, but adding them in later shouldn't be too hard. A screenshot of the particle engine to be used is below.
The code for the engine is a little bulky because of things in there that aren't really needed, so some pruning is in order. I would like to have the color of the particles be whatever color the playing piece was so that it looks like the piece itself exploded.
LANChat
See Latest Updates
Has this been done already? Yes. But my job is to unwrap what's already been done and add to it whatever a customer would need for their particular application.
Right now, I am researching various client/server architectures and collecting sample code. There are many pieces of code that I would like to include in a single project. I'd like to have the ability to have normal peer-to-peer chat (private messaging) and a "conference room" type, where all people in the public room receive all messages that anyone else in the room might send.
A future addition would include some kind of virtual blackboard, where people can draw diagrams and have them posted. However, using just a mouse and keyboard might become a little unwieldy, so this might have to be incorporated with a stylus pad. This would probably be something that only a company would invest in so that everyone could have that ability, but since I have an old pad lying around someplace, the plan is to include that function, and just shave it off if the customer doesn't want it.
Updates
12-12-08
I'd like to take a moment to describe more what I'm trying to do.
There are basically 3 different ways to communicate:
- UDP
- P2P
- Client/Server
UDP is reliable since it would send the message to everyone touchable by the current computer. However, broadcast messages shouldn't really be the standard--they're mostly used for determining where someone is on the network. It's like walking into a crowded room and shouting someone's name that you're trying to track down... and then continuing to shout a conversation back and forth once you find the person in the room. It's impolite to force everyone to listen to your conversation while they're trying to talk amongst themselves. In computer terms, software-wise, each machine has to decide whether to keep or toss every UDP packet that comes in based on who it's meant for. Lots of overhead, not a very good idea.
P2P, or Peer-To-Peer, is a direct line of communication that resembles walking over to the person you just hollered for and talking to them in a normal tone of voice. There's a definite strength to this in the amount of network noise drastically decreasing. However, the only way to sign on and make yourself recognized to (and to recognize) the people that are running the chat program is to blast off a UDP packet and waiting for the response from all computers signed on. Also, a UDP packet would have to be sent out when a person signs off so all "survivors" can update their list of online contacts. It's workable, but still kind of sloppy because somehow, computers that aren't even concerned with this chat program will be getting packets they can't use and will end up ignoring.
I'm looking for zero overhead in this respect.
The final protocol is the Client/Server protocol. This has the best of both worlds because each machine is talking to a full-time running server instead of a computer. The only thing that would have to be known in order to prevent ANY use of UDP packets is the location of the server.
That's all.
How easy is it for the server to just listen and relay any messages that come in for an intended recipient? Fairly. The server listens for any incoming messages, be it ones that are for signing in or out, or for messages meant for other computers. The server would maintain the locations of all machines--if you send a message to JoeBob123, the server would lookup the location of that machine, see that it's at address 192.168.1.204, and send the message off. That operation would be transparent to both users. Also (and this is the cool part), the only places that packets would be sent are places the server knows are online. Every type of message would be sent to the server, which relays them to the destination. And, relaying to multiple recipients would be easy too as long as the incoming message has a list of the intended targets.
Now, for some extra stuff, it would be nice if the entire system wasn't limited to just one server. This creates a major bottleneck that would stop any messaging if that server were to quit. Multiple servers would be nice, even if it's as little as two. Making sure that the server received a message by replying with an acknowledge packet would allow the client to possibly pick a different server to try to send the message again.
For larger networks consisting of smaller subnets, it would be possible to have at least one server on each subnet. Messages that are to computers on the same subnet would be routed by the server on that subnet. Messages that are to computers on different subnets would be routed by the server to the server on the desired subnet. A way to think of this would be to comparing it to how a post office works. When you mail a letter to someone in your town, the first stop is the post office, then to the recipient's house. If it's to someone out of town, the post office sends the mail to that town's post office, and that post office sends it to the recipient.
This method would cut down even more on unnecessary network traffic and really streamline code. Plus, the code itself would have to have a way of determining whether a recipient was on the subnet or was "out-of-town" so a decision could be made of how to send the message. With a client/server method, that work is up to the server.
There's a lot more I could talk about, but rather than talk myself into a corner and finding out something isn't possible, I'd rather leave things like this. Coding will begin soon for a simple client/server system.
Terramancer (hiatus)
See Latest Updates
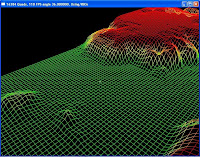
This program was used in conjunction with a freeware program called TerraGen. TerraGen was used to create the actual bitmaps, and gives free reign of how the terrain will look. A user can:
- pick how mountainous or flat the region is
- choose the size of the finished bitmap
- pick the density of various landscape features
The file is then fed into the Terramancer program. At this time, the only thing the program does is draw Quads of a given width and length (they're square), and the height value is given from the currrent pixel of the bitmap.
Very simple program. As I said, this is just a prototype program, and the main problem right now is that it's not smart to draw the entire terrain every time the draw() command is called--it eats up memory and CPU cycles to draw things that aren't visible at the player's current position.
To remedy this, I'll be incorporating a function as part of the terrain engine that will draw only the required terrain for a given area.
Phase 2
The next phase of this project will be to create something similar to TerraGen in that it will generate terrain based on given user criteria. However, the random aspect of TerraGen's production isn't conducive to this project--I need something that will create passable or fly-over-able terrain where I need it, and to have "tiles" of terrain instead of one massive terrain map. This way, I can get a tile ready for drawing, and draw smaller sections of the tile as needed.
It's hard to tell how this will be done, but it will most likely be something written in C# purely for user friendliness. A grid of squares will be given to the user, and the user can select which squares denote the passable terrain, and which areas are to be medium or high elevation. From there, the program could produce random information within given limits for different elevation types. This way, the terrain would appear more natural.
Phase 3
After this, texturing would be addressed. The terrain would look boring if not for the ability to paint portions of a bitmap onto a given polygon. Many games that include outdoor environments incorporate this in their engine, so it should be fairly easy to include this in Terramancer.
Updates
None as of yet
Minicad (revamp)
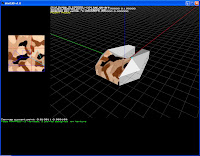
See Latest Updates
This project was created to serve as a modeling tool for various 3D games. The reason for starting this project was that I have more time and creativity than funds, and needed a way to create models in a way that doesn't milk my wallet or my time in learning how to use something complex like Milkshape or Autocad. My needs also didn't require the power that comes with any of the brand-name pieces of modeling software out there.
This project is one of the main reasons why I landed a job at Control Microsystems, because the president saw that if a tool is needed, I'd be more likely to create something custom fit than rely on something expensive that would over-solve the problem.
Right now, this project is in a revamp status. It's being revamped primarily because the user interface in C++ is pretty unwieldy. My goal is to provide the user with menu-driven buttons and clicky goodness, and it was really difficult trying to accomplish this and still maintain the flexibility I was looking for. It's my belief that porting this project over to C# to make use of that language's native user-friendliness and clean appearance, and run an OpenGL component inside to take care of the graphics aspect of this program. I've seen through the first version of this program that what I need can be done, so the experiment portion of this project is done. Now it's time to mop up the mess and make it look pretty.
Description
This project creates a model out of primitive polyhedra, custom polygons and model manipulation. I've used programs like AutoCAD in the past, and know what the bare minimum is that I'd need to do what I originally set out to do. I know that just having the ability to draw boxes, balls, cones and cylinders wasn't quite enough--I also needed a way to stretch, skew or slice these primitives and manipulate their position relative to other primitives.
The result is a model file that describes how many faces the model has, followed by each face, being described by how many vertices the face has, and then the list of vertices themselves. The model is loaded into a data structure of type "object" that contains all of the information about the object. The object file also contains which texture is to be used for a particular face, and what texture coordinates (texcoords) to use at each vertex.
Phase 2
Phase 1 consists of getting the framework down for drawing the master grid workspace and a few primitives. I think that would be fairly simple once I figure out how to splice OpenGL in with C#, so I won't go into too much detail here.
Phase 2 will start with making more progress with getting buttons and menus working to make the user experience a lot smoother. For the start, I'll probably limit how many options are available, because every time another option is created, the program needs to be that much more crash proof. I don't want to focus too much on minutae until the last.
I want to have a tab devoted to texturing. Loading the desired texture into this program will give the user the ability to see what texture they selected, and click where they what the texcoords to be on the model. For more information on how this works, click the link to that blog.
Phase 3
I've got to include some kind of picking algorithm for this thing. "Picking" is what's used when a mouse is clicked at a particular place on the screen. The program decides what the mouse was hovering over when the mouse was clicked, and selects an object based on some given criteria.
A good example would be if you went to the donut shop with a friend, and pointed to a donut without giving any verbal information. You obviously can't put your finger directly on the donut, but your friend can see from where your finger is on the glass, and where your eyes are located (observation point), and get a pretty good guess at what kind of donut you want.
Picking is similar to that--the computer knows where the observation point is programatically, and what the object's position, and how the object is located and also where the mouse is currently hovering. If the user clicks, the program should be able to determine what face the user selected.
Beyond that, it is magic to me. Although I have a grasp of it, it's going to take some work.
Phase 4
This phase will incorporate animation for the models. I don't want a bunch of static models sitting around looking pretty. I need some movement.
Creating animation will be an extra addition to model creation. The following pieces of info will be added to the model file:
- Joints
- Range of motion and
- Rate of motion
Timers will be heavily used to keep track of how long to wait between frames, and to govern what changes are made to the model.
Phase 5
This is (hopefully) the final phase, and is more of a finishing touch than something huge.
Certain games use highly detailed 2D images instead of 3D models to speed up rendering. The way this is done is to create a series of animation frames that have a masking background. Masking is a process that OpenGL uses to know where is a safe place to draw whatever happens to be behind an image.
Normally, two images are used. One is the image itself with a gray background (gray is what's used as a neutral color so the user knows what's image and what's mask). A second image is a simple black and white image--black for where the image is, and white for whatever is left. OpenGL uses these images in conjunction with each other to know where it's allowed to draw over the current image, without harming the actual image you want to keep.
A good example would be having a quad textured with something like a fence, or a grate. A second image behind it wouldn't be visible unless masking were used. To mask the grate, you need a second image that is white where the holes in the grate are located, and black where the grate itself is. Then, you'd be able to see what's behind the grate.
I said all that to say all this: I want to be able to have a set of animation frames that can be loaded into memory and that can be drawn on demand depending on the player's actions. A great example is found in Blizzard's games, such as Starcraft and Diablo. Each creature or unit has a set of animation frames for every action a particular object can do. But, the position of the object affects how it will look. So for a Marine, there are frames for when he's walking, standing idle, shooting and dying--all in one position. But there are other sets of frames for when he's walking in a different direction, shooting at something somewhere else, or dying after facing a different direction.
As for the open source availability, I'll probably have some version of it available so that my entire work isn't just thrown out there. I'd like to work on donations for this project because it would be pretty useful for people who want to snap out something simple for modeling, and can't afford thousands of dollars worth of software to do it.
Updates
None as of yet
ASP.NET/C# Network Project (future project)
This project is going to be listed as a future endeavor--not so much because I don't have time to do it right now, but because I don't know much about ASP.NET yet.
With the job market the way it is, someone with my skillset needs to have at least 1 year of exposure to ASP, and I simply don't have it. So I've got to sit down and learn it, then apply it so I can show people that I do have a working knowledge of the language.
ASP.NET's job is to create web page content quickly and dynamically. Dynamic is the way to go if you're not sure the content is always going to stay the same, and in computer languages, flexibility is a hotly desired trait.
Anytime a computer has the ability to change something without human interaction, it removes much of the work done by a programmer. Basically, the programmer builds a "machine" that is self-correcting, getting its information from an outside source to operate on, and this makes the programmer's job not so much a creation, but minor tweaks here and there on the machine's base operation.
Anyway, once I get a fairly stable working knowledge of the ASP.NET language, I'll start with some example code and applications. Like I say in my bio, I don't like to reinvent the wheel. What my normal operation is for new stuff is:
- Figure out the basics
- Find a wide variety of example code OR find a place that hosts these examples
- Get a good idea what each example does
- Select examples that either do, or almost do, what I'm looking for
- Strip off whatever code isn't useful and mix in the useful parts from the other examples
- Profit
Eventually, what I'd like to do is find a way to make ASP.NET work within a C# shell. That way, even the dynamic capability of ASP.NET can be handled dynamically by C#, resulting in a fully automated webpage updating scheme. C# and ASP.NET are high level languages, and from examples I've seen, they can tie in together.
The final application could be used in a real world scenario, but it's going to be more geared toward just familiarizing someone with ASP.NET.
Symbiosys (new project)
See Latest Updates
This project was just thought up less than a week ago. As of 12-1-08, I'm serious about starting it.
The goal of this project is to provide real-time backup of essential files. I'll be the first to admit that I don't back up anywhere near as much as I should (read: never), and I'd like a solution that doesn't force me to have to copy every single file across a network--or even to another drive--all in one shot. On top of how long it takes to copy gigabytes worth of info, I know that I'll be copying files that haven't changed since the last backup, and that's a waste of time.
"Symbiosys" was chosen as a name because of the idea of one computer on a network having room for a few computers elsewhere to place backups. Any computer running Symbiosys would serve as a place for multiple machines to store their critical files. If anything happens, any of those machines would serve as possible places to restore their files. In a sense, this is a network-oriented RAID array.
The real-time aspect of backup lets a user not worry about anything except marking which directories contain files that would need to be kept. When a file is created or changed, and that file falls within one of the directories, the file will be enqueued for backup.
Research
My research has shown me quite a few helpful things. The following things are possible in C#:
- Ability to find when files are created, accessed or changed
- Ability to choose certain directories for some purpose later on
- Copying files from one drive to another, one computer to another, or across the internet
- Obtaining attributes about a file, such as filename, location, size, last access date, etc.
- Ability to schedule when events happen using timers (fairly simple)
- Making the program run in the background, visible in the system tray in Windows XP
- How to make a program run on startup
- How to change the icon in the system tray to reflect program status
- How to find out if a workstation is in use based on monitoring mouse movement or keypresses
The operation of the program is going to be to allow a user to select certain directories that need to be backed up occasionally. The entire drive doesn't need to be backed up since things like the operating system can be reloaded in the event of a crash. The first phase isn't meant to be a total backup--rather just a way of housekeeping various files that would be problematic if there weren't any spare copies of them lying around.
The program will also ration out how much copying will be done any given time. For this description, the limiting factors will be:
- How often copying will occur
- The maximum amount of data to move at that time
Operation--Second Phase (unofficial)
The second phase, which may or may not be adopted into the program, is going to be geared toward networks that have a hard-disk server. One drive in the server will be used for holding an exact image of a drive on the network. If the primary drive crashes, the backup drive can be placed without sacrificing much time reinstalling software.
Secondary Objectives
I'd like to be able to have an Administrator version of the program that allows the change of certain settings on client machines. The Admin program would run on an independent computer on the network, and manage certain attributes, such as marking directories that the user didn't think to mark, changing the scheduling/data rate ratio, and whatever else is necessary. Since the first version of this program will be for my own benefit, development on a networking scale that would use an Admin function will be held off for now.
Updates
12-08-08
Got encryption done, and it works in favor of the program. I plan on using a key supplied with each copy of the program as the key for encryption, so that each machine with Symbiosys installed can have a unique encryption scheme for their files.
The advantage is that if something happens to the original machine's drive, and Symbiosys is wiped off, the key is still maintained with the hard copy of the program. Encrypted files will still be retrievable after Symbiosys is reinstalled.
Also, I want to give the option to NOT encrypt files, because encrypting them will generate a small amount of overhead. If someone's not really keen on that, and doesn't care that their files are visible, then the option should be available. The key will still be installed for uniqueness of copies, but just won't be used.
Network copying still isn't done yet, but it's imminent, so stay tuned.
12-05-08
Not much of an update this time, but it's still progress nonetheless.
The code now has the ability to copy a file to somewhere else on the same drive, which is what my goal was in the last update. The trick was to get the copy to maintain the exact same directory tree--not to just copy the file from one place to another.
The reason for this is simple: I want a folder somewhere (not necessarily on the same drive, but an external drive someplace) that has a name having to do with the original user's files. So if I have a directory called "Symbiosys_Backup", then in that directory have one that signifies whose files are contained in it. Inside that directory are all the directories and files that user picked to have saved.
So for the next update, I'd like to have the ability to send files across the network to another machine. It might be awhile until then since I'm not familiar with file transfer in that fashion. Hopefully it's fairly easy.
As an extra, I'd like to find a way to password-protect, or even encrypt, folders created on another machine. It's not really a needed option, but for extra security measures so that another person can't mess with an owner's files. It's worth looking into anyway.
Have a great weekend!
12-04-08
I'd like to eventually put all the different "thingies" in separate forms which will pop up when a user presses a button, or makes a menu selection. Organizing a program like what you see to the left just makes messes. TabControls aren't much better. I'm trying to stay away from information overload.
Originally the directory selection worked in a separate form, but I couldn't quickly sort out what variables could be accessed safely between forms. Now that it's laid out it's just a matter of delicate surgery, copying and pasting code where it needs to be.
The next step should be easier: The ability to copy the files that are tagged to another location. For now, it'll be somewhere on the same drive--not ideal for the final version but just to test the copying ability it'd be a good start. Also, there needs to be a way to weed out duplicate entries to prevent a file being copied multiple times, which will be part of the next update.
12-03-08
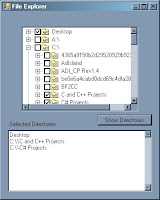
Resume
Michael G. Fritzius
2108 Freckles Drive; High Ridge, MO 63049
Cell: (636) 692-1426
code_monkey@slashmail.org
2108 Freckles Drive; High Ridge, MO 63049
Cell: (636) 692-1426
code_monkey@slashmail.org
Skill | Proficiency | Years Experience |
---|---|---|
C | Expert | 2+ |
Bash/Perl/Python Scripting | Intermediate | 2+ |
C++ | Expert | 2+ |
OpenGL | Expert | 2+ |
Java | Intermediate | 2+ |
C# | Intermediate | 2+ |
SQL and ASP | Beginner | <1 |
Ruby | Beginner | <1 |
Other Skills:
· Technical Writing
· Customer Management Skills
· Electronics Assembly and Soldering
· Experienced computer assembly (10+ years)
· Network Programming
· CAT5 Wire Construction
· SMTP and POP3 Mail operation
· Development in C and C++ in a Unix/Linux environment using makefiles and gcc
(2+ years)
· Hardware-to-PC Interface for Data Analysis
· Microsoft Word and Excel usage
· Network Packet Analysis using Wireshark
Work History:
Exegy Inc.
349 Marshall Ave-Suite 100--St. Louis, MO 63119
May 2008 to Present
Full time employment--Quality and Performance Engineer for ticker plants geared toward high frequency trading. Job includes running data quality checks to ensure that any changes to operation are either known and expected, or are unexpected and are actually bugs. Position requires an overarching knowledge of the entire system, from the data leading into the device, internal processing, and what to expect on the client side. Created tools to help in this respect to cut down on the time it takes to run the tests themselves, along with the time taken to analyze test results. These tools were mainly scripts written in Bash or Perl, or occasionally Python, and these tools' jobs were to post-analyze output created by the original testing utilities.
Control MicroSystems
405 Main Street--Fenton, MO 63026
October 2006 to May 2008
Full time employment--Lead programmer for embedded projects (microchips) covering a wide array of applications: stationary and handheld wastewater treatment modules, fuel sensors and cellular modem transmitters. Also created joint applications that interface microchips with computers for fast data transfers to free up resources from the device level. Programming languages used were:
·C for all embedded development (microchip-level programming)
·Java for some graphic interfaces concerning fuel level sensors
·C# for all graphic interfaces and front-end/back-end applications for customers
Designed software for a car seat “reminder” that plays music when a child is occupying the seat and the car hasn’t moved for a short time. The device senses movement with the use of a small digital accelerometer, and can discriminate between vehicle movement and the child jumping in the seat. This project was taken on in response to summertime fatalities of children being left in vehicles. This project was also a finalist entry in the JPMA Awards in Late Summer 2008. Information regarding this project can be found at www.carseatmonitor.com. Code for the device itself was written in C, with some analysis software being written in C#.
Developed software for devices used in storm water monitoring systems. These devices detect the amount of particulate matter in runoff, and alert the customer to send a clean-up team. This solution was put in place to save money--instead of sending a clean-up team at scheduled intervals, they can be sent when needed. The information is sent from the remote devices through a cellular modem in SMS (text message) format to a main email address. A GUI (written in C#) then intercepts and processes these messages and sends alerts depending on what information has been received. Currently, both the remote devices and the GUI are under testing and it is foreseen that up to 1,000 units are to be sold annually. These devices feature code written in C.
Designed and tested a handheld sludge detector for use in sewage treatment plants. The sludge must be monitored regularly to ensure that a backup does not occur. This device is meant to replace the clear core-sampler tubes, which are used to measure where the sludge blanket is by pulling up a vertical cylindrical sample of the fluid in the treatment tank. These tubes are not only unwieldy (being up to 16 feet long), but also become brittle with use and can snap in the wintertime--so a different solution was presented. The handheld unit is portable and battery powered and uses an infrared emitter-receiver pair, along with a pressure sensor, to determine at what depth the sludge blanket is located. The code for these pieces was also written in C.
Created and tested a wide variety of device-to-PC GUIs for use in data processing--the most recent being used for the car seat reminder described above. The basic design of these GUIs is two-pronged: the first phase is devoted to collecting raw data, while the second is a “playback” mode, showing the data on-screen as if it were real time, making debugging and algorithms much more efficient. All of these programs were created using C#, and were central to the final stages of device development.
Programmed a passive fuel level sensor for Chrysler Corporation to replace the classic “floating ball” mechanical version. Also modified a previously designed GUI written in Java that interfaced the sensor with a PC to provide examination of operation of the sensor, which made it possible to come up with a better decision algorithm. The sensor has built-in “slosh detection” to prevent false decisions due to moving fuel. Also tested in a variety of temperatures to make sure the sensor would operate normally under all conditions. The code for these sensors was written in C.
Designed software in C# for a multiple solenoid timer tester (up to ten timers simultaneously) that communicates with a PC via RS232 cable. The software receives information from a testing board and determines if the timers are switching on and off at the appropriate times. It allows a user to pick a tolerance of values around the target times for each event.
Currently developing an aftermarket field camera modification for hunters that can transmit its payload of pictures to an email address, whose processor is loaded with code written in C. This device “piggybacks” on the camera and controls access to an SD card while the camera is in an idle state. Information is retrieved from the SD card by using the FAT16 file system. The device then initiates an internet connection via cellular modem. Emails can be sent to any destination using the SMTP protocol, which includes the desired picture encoded in the attachment section of the email per RFC 822 protocol. The end result is an email sent to the customer containing the most recent picture.
Programmed and tested a marine application that uses a digital gyroscope to perform load control on open water. The device will let a user know when the load has shifted, giving warning for correction. This device is currently in prototype status. The code for this project is written in C, with a C# graphic interface being used for initial algorithm testing.
Developed a controller for a customer whose business involves hardware recovery of devices submerged accidentally or become waterlogged from firefighting. This controller will combine and replace many independent controllers aboard the current model, and has been offered as a lower-cost solution—both in time spent constructing the units, and overall cost. This project is in the prototype stage, but full-scale production is imminent. The code is written in C for this project.
Schnucks Supermarkets
1393 Big Bend Road--Suite 1
Ballwin, MO 63021
October 2001 to April 2008
Part time employment—Customer Service, catering, face-to-face and over-the-phone customer interaction, various maintenance duties.
Current Projects
A miniature version of AutoCAD that will be used in upcoming game projects whose goal is to facilitate the creation of 3D objects and models. The entire project is currently written in OpenGL based in C++. This program handles texturing/skinning operations, “smoothing” algorithms, allows the user complete control over model viewing, and will evolve to handle model animation. The final release will be open source and will be ported to C# for ease of use.
Learning ASP.NET operation, familiarizing myself with its use, and how it can be used to modify web pages with new information. To speed the learning process, some sample C# programs will be created that have access to the same web page, and that can read/modify the page’s content.
A backup scheduling program called Symbiosys that allows a user to backup files within marked folders. Symbiosys differs from most other backup software in that it only works on files that have been changed since the last backup. It will also allow a user to backup files to a local disk, a network drive, or even a remote server. This program will run in the background monitoring which files have been changed, and begin a backup at a time with minimal system usage. Many features will be added as the program grows and feedback is obtained during testing. This program is written in C#.
A simple puzzle game written in C++ using OpenGL for graphics. Currently, the game engine is under development. Extra features include a particle engine based in C++/OpenGL, and sound and music using the fmod library. The game and graphics engines will be used in similar projects in the near future.
These and other projects can be found in greater detail at http://www.technomancer-michael.blogspot.com.
Education:
University of Missouri—St. Louis
Bachelor of Science, May 2006, Computer Science—Minor in Philosophy, Mathematics
Jefferson College—Hillsboro, Missouri
Electronics Certificate, A.A.S. Industrial Automation, May 2003